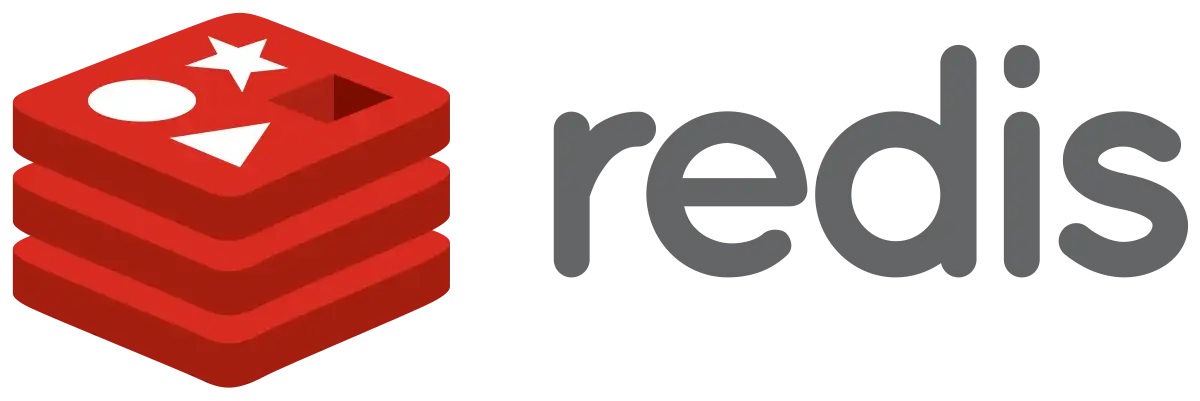
Redis入门到精通
2022-10-05 21:03:06
2025-05-22 02:56:25
客户端下载
官方地址
http
https://redis.com/redis-enterprise/redis-insight/#insight-form
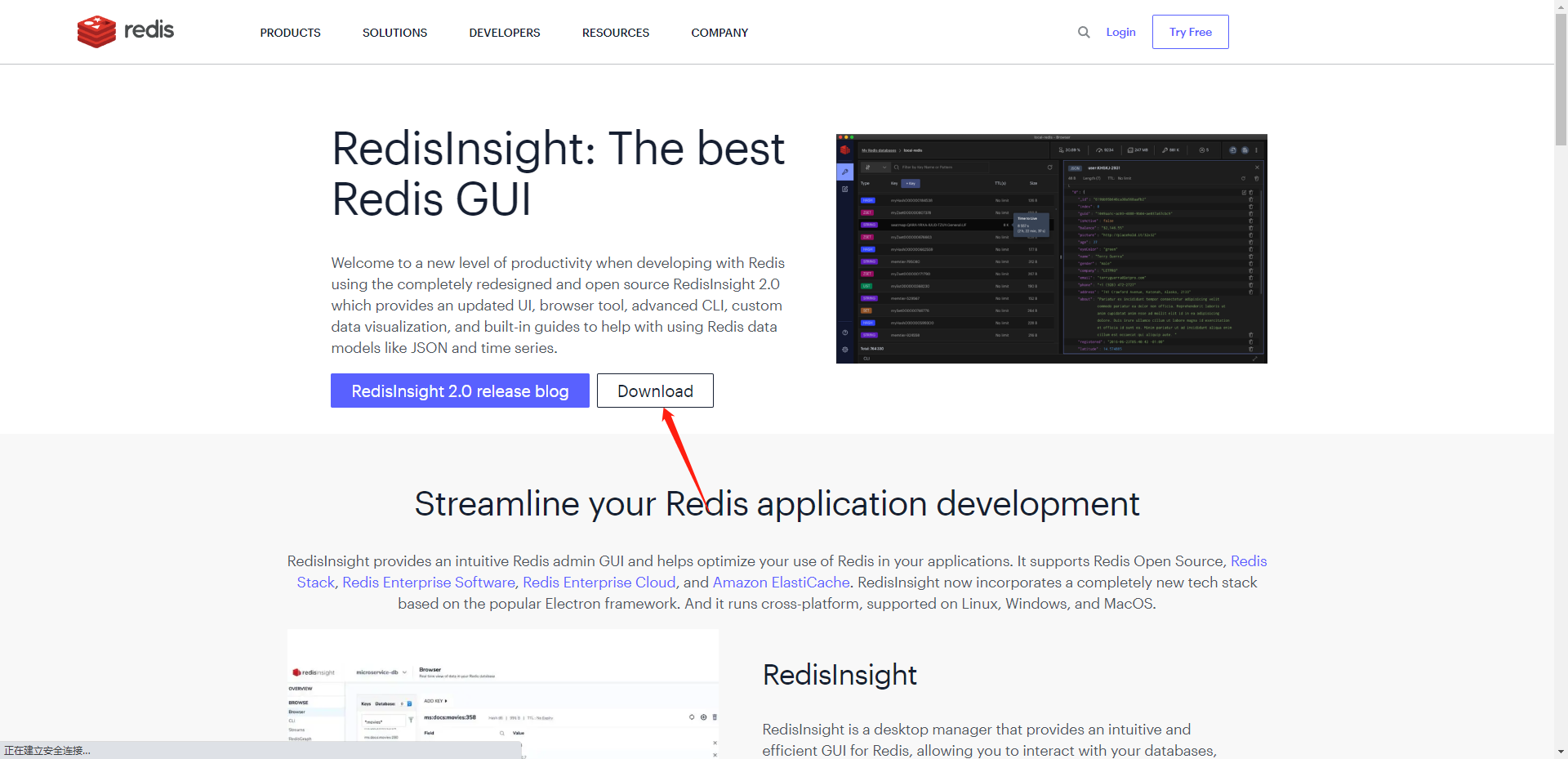
redis封装
shell
pnpm add -S ioredis
js
import { Logger } from '@nestjs/common';
import Redis from 'ioredis';
const logger = new Logger('auth.service');
const redisIndex = []; // 用于记录 redis 实例索引
const redisList = []; // 用于存储 redis 实例
const redisOption = {
host: 'IP地址',
port: 6379,//端口号
password: '密码',
};
export class RedisInstance {
static async initRedis(method: string, db = 0) {
const isExist = redisIndex.some((x) => x === db);
if (!isExist) {
Logger.debug(`[Redis ${db}]来自 ${method} 方法调用 `);
redisList[db] = new Redis({ ...redisOption, db });
redisIndex.push(db);
} else {
Logger.debug(`[Redis ${db}]来自 ${method} 方法调用`);
}
return redisList[db];
}
static async setRedis(method: string, db = 0, key: string, val: any, timeout = 60 * 60) {
if (typeof val == 'object') {
val = JSON.stringify(val);
}
const redis = await RedisInstance.initRedis(method, db);
redis.set(`${key}`, val);
redis.expire(`${key}`, timeout);
}
static async getRedis(method: string, db = 0, key: string) {
return new Promise(async (resolve, reject) => {
const redis = await RedisInstance.initRedis(method, db);
redis.get(`${key}`, (err, val) => {
if (err) {
reject(err);
return;
}
resolve(val);
});
});
}
}
Redis使用
js
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Navigation } from './entities/navigation.entity';
import { CreateNavigationDto } from './dto/create-navigation.dto';
import { UpdateNavigationDto } from './dto/update-navigation.dto';
import { RedisInstance } from 'src/utils/redis';
@Injectable()
export class NavigationService {
constructor(
@InjectRepository(Navigation)
private readonly NavigationModel: Repository<Navigation>,
) {}
async create(params: CreateNavigationDto) {
return await this.NavigationModel.save(params);
}
async update(params) {
//判断是不是数组
if (!Array.isArray(params)) {
params = [params];
}
params.forEach(async (item) => {
return await this.NavigationModel.createQueryBuilder()
.update(Navigation)
.set(item)
.where('id = :id', { id: item.id })
.execute();
});
this.findAll('', true);
}
z;
async findAll(params, isUpdateCache = false) {
const { page = 1, pageSize = 1000, status, typeId } = params;
const where: any = {};
status && (where.status = status);
typeId && (where.typeId = typeId);
const rowsKey = 'navigation_rows';
const countKey = 'navigation_count';
//存储导航列表到redis
if (isUpdateCache) {
const rows = await this.NavigationModel.find({
order: { orderId: 'ASC' },
where,
skip: (page - 1) * pageSize,
take: pageSize,
cache: true,
});
const count = await this.NavigationModel.count({ where });
await RedisInstance.setRedis('navigation', 0, rowsKey, rows);
await RedisInstance.setRedis('navigation', 0, countKey, count);
} else {
//查询全部数据,走缓存
if (page === 1 && pageSize === 1000 && JSON.stringify(where) === '{}') {
const rowsRedis = (await RedisInstance.getRedis('navigation', 0, rowsKey)) as string;
const countRedis = (await RedisInstance.getRedis('navigation', 0, countKey)) as string;
//如果两个都为空就重新查询一次
if (!rowsRedis && !countRedis) {
const rows = await this.NavigationModel.find({
order: { orderId: 'ASC' },
where,
skip: (page - 1) * pageSize,
take: pageSize,
cache: true,
});
const count = await this.NavigationModel.count({ where });
await RedisInstance.setRedis('navigation', 0, rowsKey, rows);
await RedisInstance.setRedis('navigation', 0, countKey, count);
return { rows, count };
}
return { rows: JSON.parse(rowsRedis), count: countRedis };
} else {
//不走缓存
const rows = await this.NavigationModel.find({
order: { orderId: 'ASC' },
where,
skip: (page - 1) * pageSize,
take: pageSize,
cache: true,
});
const count = await this.NavigationModel.count({ where });
return { rows, count };
}
}
}
async remove(ids) {
const arr = ids?.split(',');
const res = await this.NavigationModel.createQueryBuilder()
.delete()
.from(Navigation)
.whereInIds(arr.map((item) => Number(item)))
.execute();
this.findAll('', true);
return res;
}
}
目录